DEVELOPER API DOCUMENTATION
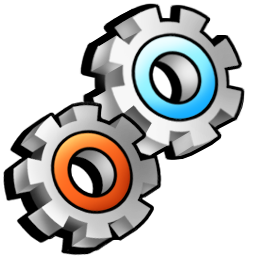
This page is for software developers who want to integrate the Indexification API into their own software or service!
Integrating our API into your products will only benefit You and Your customers for even more Automation,
time saving and ease of use!
The Indexification API provides an easy interface for programmers to embed Indexification functions in their
own programs or online services directly. The Indexification API is very easy to use - it uses
HTTP Requests to receive Your commands, process them and return You a result.
In case You are in need of a test API Key to test Your code implementations and integration simply register Yourself
an account (it is absolutely free to register) and then
Contact Us
to set Your account to a developer one!
API URL - http://www.indexification.com/api.php
The
API URL is the address which You must use to communicate with our API
POST Method Only
You Must use
HTTP POST Method only when communicating with our API
AVAILABLE VARIABLES
apikey - This is an API Authentication Key generated by our system for each single client. It is used to authenticate
as the corresponding user. Every our customer can find his API Key inside the members area at our site.
remaininglimit=1 - Use this variable to return the Remaining daily links limit.
campaign - A campaign name under which the URLs sent will be assigned. Campaigns are used in our system to help
out customers organize their submitted URLs by groups. If this variable is omitted or is left blank our system will create a campaign
with current date time stamp. If a campaign with the name provided already exists, our system will automatically
add some random characters to the end of it.
dripfeed - Specifies Scheduling options. There are 3 possible values.
XX_days - Drip feed all links within XX days,
XX_links - Drip feed at XX links a day,
disabled - Submit All Links ASAP (No Dripfeeding).
Ofcourse replace
XX with your desired values.
If this variable is omitted or left blank our system will set a default value of
Drip feed all links within 7 days
Example:
dripfeed=10_days will make all links dripfeeding within 10 days.
Example:
dripfeed=100_links will make all links dripfeeding with a 100 links a day rate.
Example:
dripfeed=disabled will mark all links for immediate processing.
urls - This is a string collection of all URLs that will be sent to our system. Delimiter between
each URL is | (single pipe) without any spaces or new lines.
If more links than the available user's remaining daily limit are tried to be submitted, our system will accept
only that much as the remaining limit is!
Please send all URLs at once, in one call at the end of your link building session. Not one or few URLs per call.
QUERY TYPES
There are two Query Types available:
1. Remaining Limit - For this Query type You use only
apikey and
remaininglimit variables
2. Links Submission - For this Query type You use
apikey, campaign, dripfeed, urls variables.
API RESPONSES
###### - Returns the User's Remaining Daily Limit, where ###### is the actual limit number
OK - Returned if everything went smooth on a Links Submission Query
ERROR: xxxxxxxxx.....xxxxxx - Returned upon encountering an error. Description of the exact error is displayed as well
COPY & PASTE CODE EXAMPLES
PHP (Retrieve Remaining Daily Links Limit)
<?php
$apikey='YOUR_API_KEY_HERE';
// build the POST query string
$qstring='apikey='.$apikey.'&remaininglimit=1';
// Do the API Request using CURL functions
$ch = curl_init();
curl_setopt($ch,CURLOPT_POST,1);
curl_setopt($ch,CURLOPT_URL,'http://www.indexification.com/api.php');
curl_setopt($ch,CURLOPT_HEADER,0);
curl_setopt($ch,CURLOPT_RETURNTRANSFER,1);
curl_setopt($ch,CURLOPT_TIMEOUT,40);
curl_setopt($ch,CURLOPT_POSTFIELDS,$qstring);
$response=curl_exec($ch);
curl_close($ch);
echo $response;
?>
PHP (Links Submission)
<?php
$apikey='YOUR_API_KEY_HERE';
$campaign='CAMPAIGN_NAME_HERE';
// All URLS to be sent are hold in an array for example
$urls=array('http://www.site1.com','http://www.site2.com/');
// build the POST query string and join the URLs array with | (single pipe)
$qstring='apikey='.$apikey.'&campaign='.$campaign.'&urls='.urlencode(implode('|',$urls));
// Do the API Request using CURL functions
$ch = curl_init();
curl_setopt($ch,CURLOPT_POST,1);
curl_setopt($ch,CURLOPT_URL,'http://www.indexification.com/api.php');
curl_setopt($ch,CURLOPT_HEADER,0);
curl_setopt($ch,CURLOPT_RETURNTRANSFER,1);
curl_setopt($ch,CURLOPT_TIMEOUT,40);
curl_setopt($ch,CURLOPT_POSTFIELDS,$qstring);
curl_exec($ch);
curl_close($ch);
?>
VB.NET using Web Client (Links Submission)
Dim Apikey As String = "YOUR_API_KEY_HERE"
Dim Campaign As String = "CAMPAIGN_NAME_HERE"
Dim Urls() As List(Of String) = {"http://www.site1.com","http://www.site2.com/"}
Dim Qstring As String = "apikey=" & UrlEncode(Apikey) & "&campaign=" & UrlEncode(Campaign) & "&urls=" & UrlEncode(Join(Urls.ToArray, "|"))
Try
Dim wb As WebClient = New WebClient
wb.Proxy = Nothing
wb.UploadString("http://www.indexification.com/api.php", "POST", Qstring)
Catch ex As WebException
End Try
VB.NET using pure HTTP Web Requests (Links Submission)
Dim Apikey As String = "YOUR_API_KEY_HERE"
Dim Campaign As String = "CAMPAIGN_NAME_HERE"
Dim Urls() As List(Of String) = {"http://www.site1.com","http://www.site2.com/"}
Dim Qstring As String = "apikey=" & UrlEncode(Apikey) & "&campaign=" & UrlEncode(Campaign) & "&urls=" & UrlEncode(Join(Urls.ToArray, "|"))
Try
Dim myHttpWebRequest As HttpWebRequest = CType(WebRequest.Create("http://www.indexification.com/api.php"), HttpWebRequest)
myHttpWebRequest.AllowAutoRedirect = True
myHttpWebRequest.Timeout = 40000
myHttpWebRequest.ReadWriteTimeout = 40000
myHttpWebRequest.Method = "POST"
myHttpWebRequest.ContentType = "application/x-www-form-urlencoded"
Dim postBuffer As Byte() = System.Text.Encoding.UTF8.GetBytes(Qstring)
myHttpWebRequest.ContentLength = postBuffer.Length
Dim requestStream As Stream = myHttpWebRequest.GetRequestStream()
requestStream.Write(postBuffer, 0, postBuffer.Length)
requestStream.Flush()
requestStream.Close()
requestStream.Dispose()
Dim myHttpWebResponse As HttpWebResponse = CType(myHttpWebRequest.GetResponse(), HttpWebResponse)
Dim streamIn As StreamReader = New StreamReader(myHttpWebResponse.GetResponseStream())
streamIn.ReadToEnd()
streamIn.Close()
myHttpWebResponse.Close()
Catch e As WebException
End Try
C++ using Web Client (Links Submission)
string Apikey = "YOUR_API_KEY_HERE";
string Campaign = "CAMPAIGN_NAME_HERE";
List[] Urls = {
"http://www.site1.com",
"http://www.site2.com/"
};
string Qstring = "apikey=" + UrlEncode(Apikey) + "&campaign=" + UrlEncode(Campaign) + "&urls=" + UrlEncode(Strings.Join(Urls.ToArray, "|"));
try {
WebClient wb = new WebClient();
wb.Proxy = null;
wb.UploadString("http://www.indexification.com/api.php", "POST", Qstring);
} catch (WebException ex) {
}
In case You are in need of a test API Key to test Your code implementations and integration simply register Yourself
an account (it is absolutely free to register) and then
Contact Us
to set Your account to a developer one!
Having problems implementing Your code to use with Our API? You discovered a bug in our API?
Contact Us
Don't forget to Contact Us once You integrated our API
into Your software or online service and do some link exchanges and cross promotion of our products for even
bigger audience!